Drive Manager#
The DriveManager component is responsible for direct control communication with the robot. It is used to perform last checks on any control command before passing it to the robot to ensure that the commands falls within the robot control limits, satisfies smoothness conditions and does not lead to a collision with a nearby obstacle.
The DriveManager component can perform one or multiple of the following functionalities based on the desired config:
Emergency Stopping |
Checks the direct sensor information from the configured proximity sensors and performs an emergency stop if an obstacle is within the conical emergency zone configured with a minimum safety distance (m) and and angle (rad) in the direction of the robot movement. |
Emergency Slowdown |
Similar to the emergency zone, a slowdown zone can be configured to reduce the robot’s velocity if an obstacle is closer than the slowdown distance. |
Control Limiting |
Checks that the incoming control is within the robot control limits. If a control command is outside the robot control limits, the DriveManager limits the value to the maximum/minimum allowed value before passing the command to the robot |
Control Smoothing (Filtering) |
Performs smoothing filtering on the incoming control commands before sending the commands to the robot |
Robot Unblocking |
Moves the robot forward, backwards or rotates in place if the space is free to move the robot away from a blocking point. This action can be configured to be triggered with an external event |
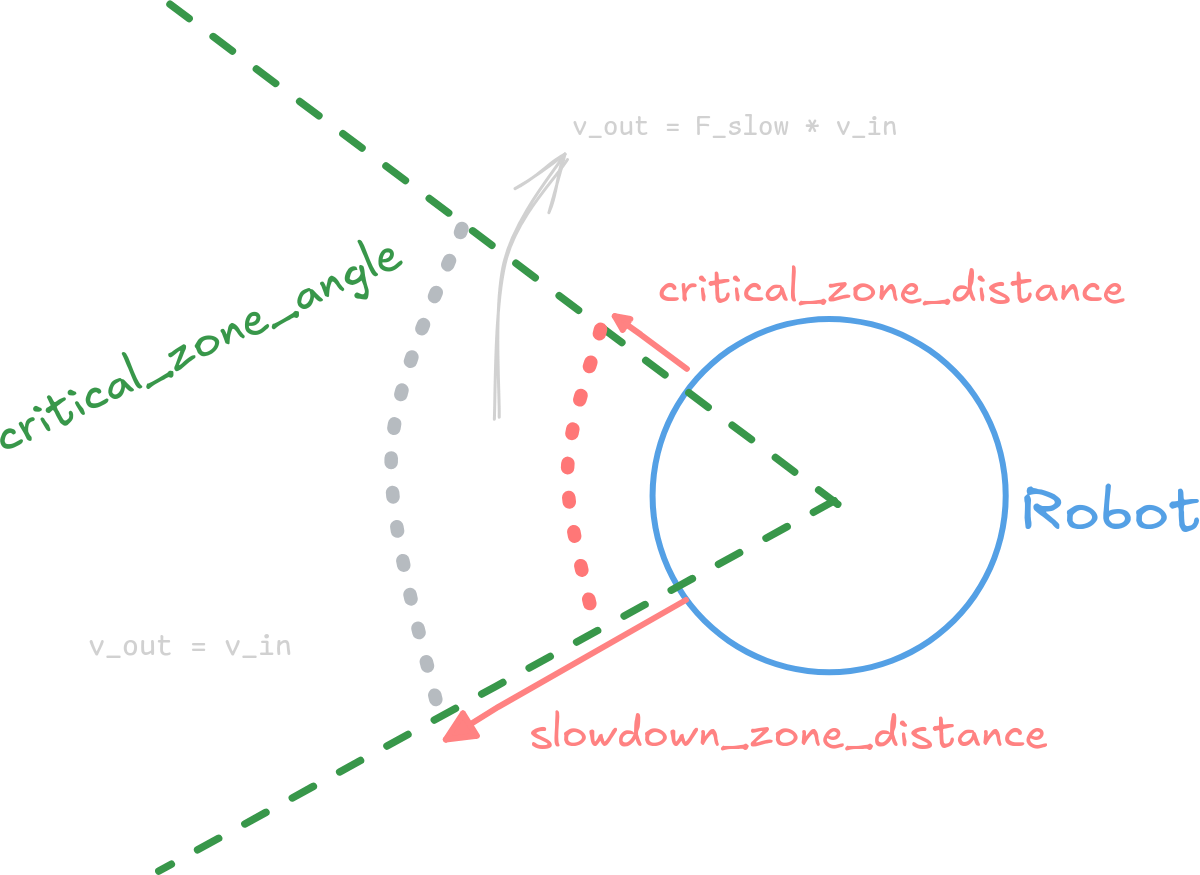
Emergency Zone & Slowdown Zone#
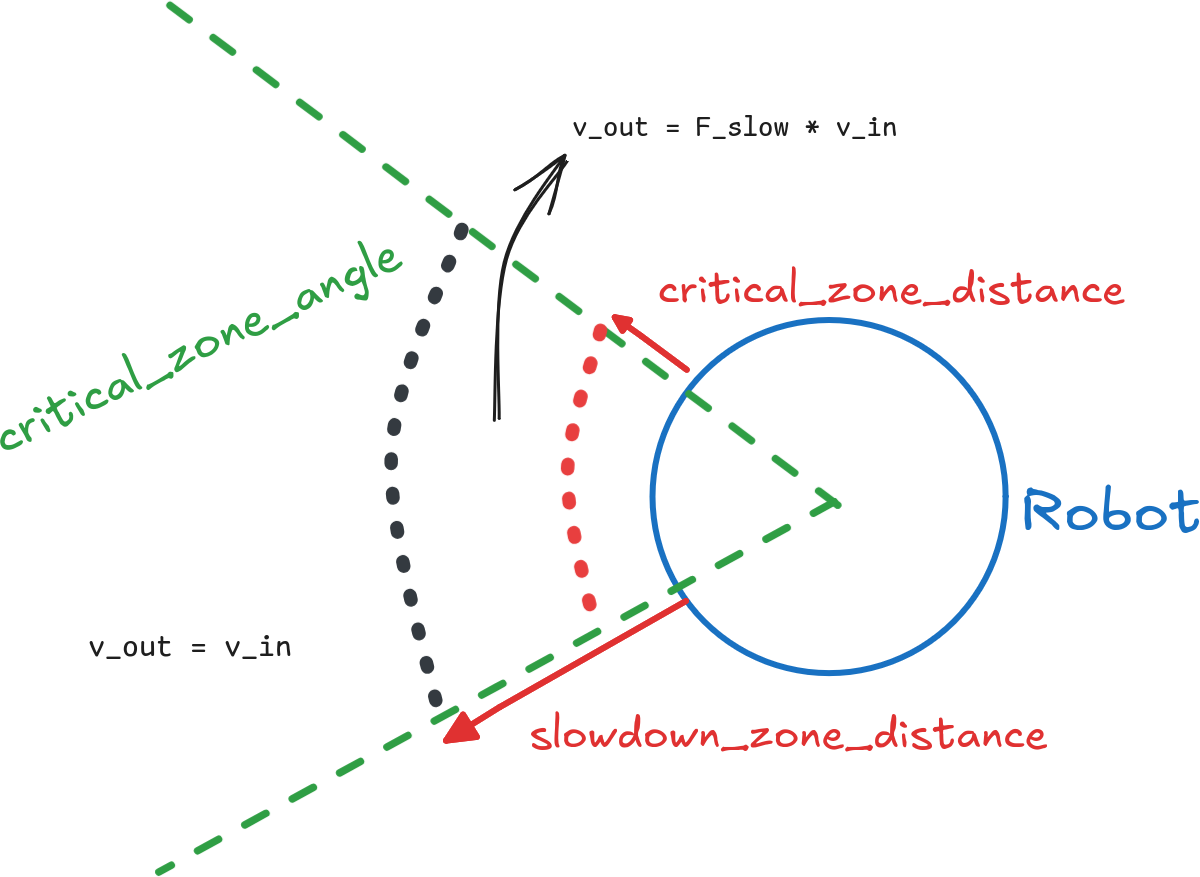
Emergency Zone & Slowdown Zone#
Note
Critical and Slowdown Zone checking is implemented in C++ in kompass-core for fast emergency behaviors. The core implementation supports both GPU and CPU (defaults to GPU if available).
DriveManager also includes built-in movement actions used for directly control the robot or unblocking the robot in certain conditions:
Action |
Function |
---|---|
Moves the robot forward for |
|
move_backward |
Moves the robot backwards for |
rotate_in_place |
Rotates the robot in place for |
move_to_unblock |
Moves the robot forward, backwards or rotates in place if the space is free to move the robot away from a blocking point. |
Note
All the previous movement actions require LaserScan
information to determine if the movement direction is collision-free
See also
Check an example on configuring the robot unblocking functionality with an external event in this tutorial
Available Run Types#
Timed |
Sends incoming command periodically to the robot |
Inputs#
Key Name |
Allowed Types |
Number |
Default |
---|---|---|---|
command |
1 |
|
|
multi_command |
1 |
|
|
sensor_data |
|
1 + (10 optional) |
|
location |
|
1 |
|
Outputs#
Key Name |
Allowed Types |
Number |
Default |
---|---|---|---|
robot_command |
|
1 |
|
emergency_stop |
|
1 |
|
Configuration Parameters:#
See all available parameters in DriveManagerConfig
Usage Example:#
from kompass.components import DriveManager, DriveManagerConfig
from kompass.ros import Topic
# Setup custom configuration
# closed_loop: send commands to the robot in closed loop (checks feedback from robot state)
# critical_zone_distance: for emergency stp (m)
my_config = DriveManagerConfig(closed_loop=True, critical_zone_distance=0.1, slowdown_zone_distance=0.3, critical_zone_angle=90.0)
driver = DriveManager(component_name="driver", config=my_config)
# Change the robot command output
driver.outputs(robot_command=Topic(name='/my_robot_cmd', msg_type='Twist'))